The Ultimate Troubleshooting Guide for Semantic Errors in Chapter 80
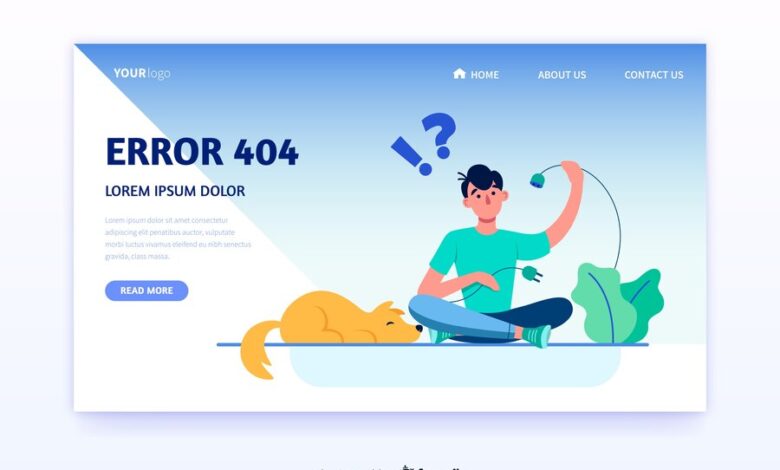
Introduction to Semantic Errors and Their Impact on Web Development
In the intricate world of web development, encountering errors is all too common. Among these errors, semantic errors are particularly challenging as they involve the logic and meaning behind your code rather than its syntax. This makes them harder to detect and solve, but also crucial to address for the functionality and performance of your applications.
Semantic errors can lead to unexpected behaviors, data corruption, and performance issues, severely impacting both user experience and business outcomes. As such, understanding and effectively troubleshooting these errors is a critical skill for web developers and programming enthusiasts alike.
This guide aims to provide an extensive, step-by-step approach to identifying, debugging, and resolving semantic errors, particularly within the context of Chapter 80—a hypothetical yet complex segment of code.
Understanding Semantic Errors: Types, Causes, and Common Mistakes
Types of Semantic Errors
- Logical Errors: These occur when the code runs without syntax issues, but the logic doesn’t achieve the intended outcome. For example, using the wrong algorithm or misunderstanding the problem requirements.
- Arithmetic Errors: Miscalculations due to incorrect operations, such as dividing by zero or using wrong precedence in mathematical expressions.
- State Errors: Issues arising from incorrect management of application state, often found in asynchronous programming or state-heavy applications like React or Vue.js.
Causes of Semantic Errors
- Misunderstanding Requirements: Incorrectly interpreting the functional requirements of a project.
- Inadequate Testing: Lack of thorough testing can allow semantic errors to slip through.
- Complex Logic: Errors often arise in complex conditional statements and loops.
- Copy-Paste Programming: Reusing code snippets without sufficient understanding can introduce errors.
Common Mistakes
- Misusing Boolean conditions.
- Incorrect variable scope or variable shadowing.
- Off-by-one errors in loops.
- Incorrect data type usage or conversion.
The Ultimate Troubleshooting Guide for Semantic Errors in Chapter 80
Step-by-step Problem Identification
- Reproduce the Issue: Start by consistently reproducing the error. This aids in isolating the part of the code causing the issue.
- Understand the Context: Review the relevant section of Chapter 80 to understand what the code is supposed to accomplish.
- Check Recent Changes: Look into recent modifications in the code base. Semantic errors often surface after new features or changes are introduced.
Best Practices for Debugging Semantic Errors
- Code Reviews: Peer reviews can catch semantic errors that automated tests might miss.
- Unit Testing: Write unit tests to check the logic of individual components, ensuring they behave as expected.
- Logging: Implement detailed logging to capture the state of the application at various points during execution.
- Step-through Debugging: Use debugging tools to walk through the code line by line and inspect variable states.
Tools and Resources for Efficient Troubleshooting
- Integrated Development Environments (IDEs): IDEs like Visual Studio Code, IntelliJ IDEA, and Eclipse offer powerful debugging tools.
- Linting Tools: ESLint, JSLint, and Pylint help in identifying potential semantic issues early in the development cycle.
- Static Analysis Tools: Tools like SonarQube and Coverity scan code for logical errors and vulnerabilities.
- Documentation and Forums: Resources like Stack Overflow, GitHub Issues, and official documentation can provide valuable insights.
Real-world Examples of Semantic Errors and Their Solutions
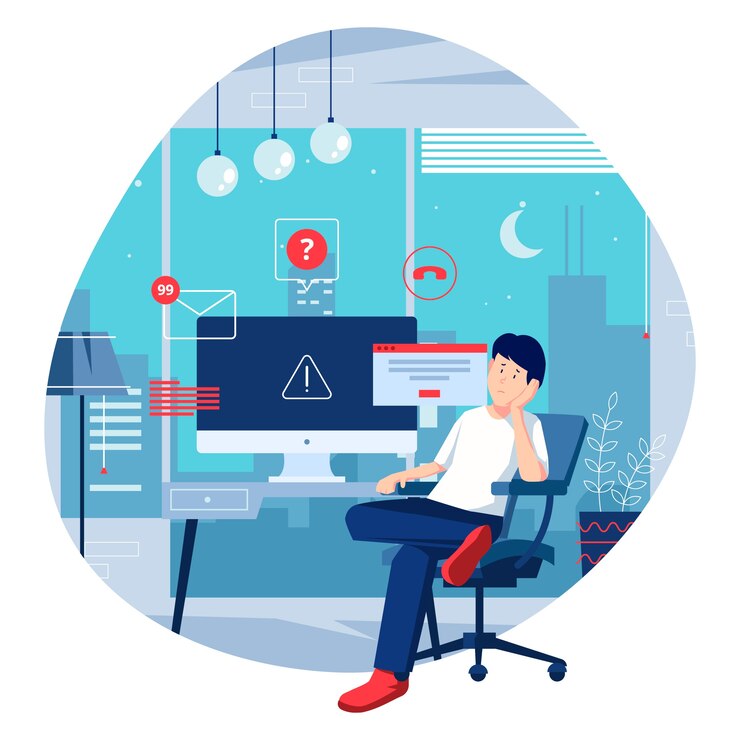
Example 1: Misuse of Conditional Statements
Problem: A function is supposed to return true if a user is an admin or a moderator, but it always returns false.
Error:
“`javascript
function isAdminOrModerator(user) {
if (user.role === ‘admin’ || user.role === ‘moderator’)
return false;
return true;
}
“`
Solution: Change `return false` to `return true`.
“`javascript
function isAdminOrModerator(user) {
if (user.role === ‘admin’ || user.role === ‘moderator’)
return true;
return false;
}
“`
Example 2: Incorrect Loop Conditions
Problem: A loop iterates one time too many, causing an overflow error.
Error:
“`python
for i in range(0, len(arr)):
process(arr[i + 1])
“`
Solution: Adjust the loop condition to avoid accessing out-of-bound indices.
“`python
for i in range(0, len(arr) – 1):
process(arr[i + 1])
“`
Future-proofing Your Code Against Semantic Errors
Adopting Best Practices
- Continuous Integration/Continuous Deployment (CI/CD): Implement CI/CD pipelines to ensure automated testing and code quality checks.
- Code Readability: Write clean, readable code with comments and documentation to reduce misunderstandings.
- Pair Programming: Collaborate with another developer to catch semantic errors early in the development process.
- Regular Refactoring: Continuously improve and refactor code to minimize complexity and improve maintenance.
Leveraging Modern Tools
- AI-powered Code Review Tools: Tools like DeepCode and Codota use machine learning to identify potential semantic errors.
- Advanced Debugging Techniques: Techniques such as time-travel debugging allow you to move back and forth in the application’s execution to understand state changes leading to errors.
Conclusion: Key Takeaways for Web Developers and Programming Enthusiasts
Semantic errors, though subtle and often elusive, can have significant impacts on the functionality and performance of your applications. By understanding their types, causes, and common pitfalls, and by employing systematic troubleshooting strategies, you can effectively resolve these issues.
Remember:
- Always reproduce and understand the error context.
- Leverage code reviews, unit testing, and logging for debugging.
- Utilize modern tools and resources for efficient troubleshooting.
- Adopt best practices to future-proof your code against semantic errors.
By mastering the art of identifying and resolving semantic errors, you not only improve the quality of your code but also enhance your problem-solving skills, making you a more proficient and reliable developer. Stay curious, keep learning, and happy coding!
For more tips and in-depth guides, sign up for our newsletter and stay updated with the latest in web development.