Mastering JavaScript Arrays Common Pitfalls to Avoid in Comparison
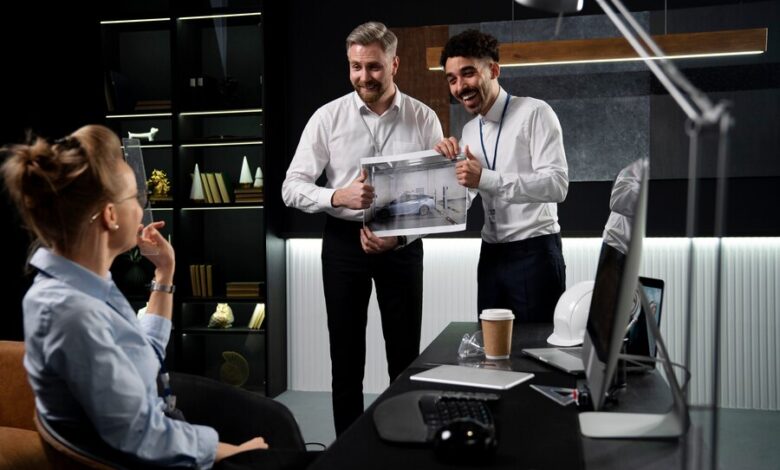
JavaScript is an essential skill for web developers. Arrays are a core data structure in JavaScript, but comparing them can be tricky. Missteps in array comparison can lead to bugs that are hard to track down. Let’s explore the common pitfalls in JavaScript array comparison and learn how to avoid them.
Introduction to JavaScript Array Comparison
JavaScript arrays form the backbone of many applications. They store multiple values in a single variable, making them incredibly useful. However, comparing arrays isn’t straightforward. Many developers encounter unexpected results because they miss the finer points of JavaScript’s comparison behavior.
In this blog post, we’ll uncover the common pitfalls in JavaScript array comparison. We’ll also discuss best practices that provide accurate and efficient comparisons. Whether you’re a newbie or a seasoned developer, this guide offers valuable insights into mastering array comparison.
Shallow vs. Deep Equality Checks
Understanding Shallow Equality
Shallow equality checks determine whether two arrays are identical by comparing their references. In JavaScript, this is done using the `===` operator. However, this only works if both arrays point to the same object in memory.
Example:
“`
const array1 = [1, 2, 3];
const array2 = [1, 2, 3];
console.log(array1 === array2); // false
“`
The Need for Deep Equality
Deep equality checks go beyond references. They compare the contents of the arrays, element by element. This is crucial when you need to confirm that two arrays have the same values in the same order.
Implementing Deep Equality Checks
You can implement deep equality checks using loops or recursion. Libraries like Lodash also offer functions like `isEqual` to simplify this task.
Example:
“`
const isEqual = (arr1, arr2) => JSON.stringify(arr1) === JSON.stringify(arr2);
“`
Null vs. Undefined Values
The Difference Between Null and Undefined
JavaScript distinguishes between `null` and `undefined`. `null` represents an intentional absence of value, while `undefined` indicates a variable that has not been assigned a value.
Pitfalls in Array Comparison
When comparing arrays, treating `null` and `undefined` as equivalent can lead to errors. It’s important to handle these values explicitly to avoid unexpected behavior.
Handling Null and Undefined
Use strict equality (`===`) to compare `null` and `undefined` separately. This ensures that your comparisons reflect the distinct meanings of these values.
Example:
“`
const array1 = [null];
const array2 = [undefined];
console.log(array1[0] === array2[0]); // false
“`
Reference vs. Value Comparison
Understanding References
In JavaScript, arrays are reference types. This means that when you assign an array to a variable, you’re assigning a reference to the array, not the array itself. Comparing references checks if two variables point to the same array.
The Limitation of Reference Comparison
Reference comparison can be misleading. Two arrays with identical values but different references will not be considered equal.
Using Value Comparison
Value comparison involves comparing the actual contents of arrays. This is more reliable for determining equality but requires more effort to implement correctly.
Performance Considerations
The Cost of Deep Comparison
Deep comparison is more computationally expensive than shallow comparison. It involves traversing the entire array and comparing each element, which can be slow for large arrays.
Optimizing Performance
To optimize performance, avoid unnecessary deep comparisons. Use shallow comparisons when appropriate and consider caching results if the same comparisons are needed repeatedly.
Built-in Methods for Performance
JavaScript’s built-in methods, like `Array.prototype.every` and `Array.prototype.some`, can help optimize performance. These methods provide efficient ways to check conditions across arrays.
Using Built-in Methods
Leveraging Array Methods
JavaScript offers several built-in methods for array comparison. Methods like `every`, `some`, and `includes` can simplify your code and improve readability.
Example:
“`
const array1 = [1, 2, 3];
const array2 = [1, 2, 3];
const areEqual = array1.length === array2.length && array1.every((value, index) => value === array2[index]);
console.log(areEqual); // true
“`
Benefits of Built-in Methods
Using built-in methods reduces the need for custom comparison functions. They are optimized for performance and often easier to understand.
Limitations to Consider
While built-in methods are powerful, they may not cover all use cases. For complex comparisons, you might still need custom logic.
Implementing Custom Comparison Functions
When to Use Custom Functions
Custom comparison functions are useful when built-in methods fall short. They offer flexibility to handle unique comparison requirements.
Writing Custom Functions
Custom functions can be tailored to your specific needs. They may involve loops, conditionals, and recursion to achieve the desired comparison.
Example:
“`
const customCompare = (arr1, arr2) => {
if (arr1.length !== arr2.length) return false;
for (let i = 0; i < arr1.length; i++) {
if (arr1[i] !== arr2[i]) return false;
}
return true;
};
“`
Testing Custom Functions
Thoroughly test your custom functions to ensure they handle all edge cases. Consider performance implications and optimize as needed.
Leveraging ES6 Features for Simplicity
The Power of ES6
ES6 introduced several features that simplify array comparison. Features like the spread operator and `Array.from` make it easier to work with arrays.
Using the Spread Operator
The spread operator (`…`) allows you to create shallow copies of arrays. This is useful for avoiding reference comparison issues.
Example:
“`
const array1 = [1, 2, 3];
const array2 = […array1];
console.log(array1 === array2); // false
console.log(array1.toString() === array2.toString()); // true
“`
Benefits of ES6 Syntax
ES6 syntax is more concise and often more readable. It can reduce the complexity of your code and make array comparisons more intuitive.
Real-World Applications and Examples
Comparing User Input
In web applications, comparing user input is common. Whether it’s form data or user preferences, accurate array comparison ensures that your application behaves as expected.
Data Synchronization
Array comparison is crucial for data synchronization tasks. Keeping client and server data in sync often requires comparing arrays to identify changes.
Testing and Debugging
During testing and debugging, array comparison helps verify that your code produces the expected results. Automated tests often rely on array comparison to validate functionality.
Conclusion
Mastering JavaScript array comparison is essential for any web developer. By understanding the common pitfalls and adopting best practices, you can avoid bugs and write more reliable code. Accurate array comparison is not just a technical skill; it’s a critical aspect of building robust applications.
Whether you’re comparing user input, synchronizing data, or writing tests, these techniques will serve you well. Keep exploring and experimenting with different methods to find what works best for your specific needs.
If you’re ready to take your JavaScript skills to the next level, start implementing these array comparison techniques today. Your future self (and your users) will thank you!